Cut2D hk0¶
After inspecting the scattering plane, we want to perform cuts along certain directions. In this tutorial, we demonstrate the cut2D function. Cuts can be made given by hkl or Qx, Qy, Qz, by using rlu=True/False. The width of the cut orthogonal to the plane can be adjusted by the keywords width. The grid the cut is projected on is given by the xBins and yBins keywords or dQx and dQy. The binning is allways given in Q-space, also when you do a cut in HKL-space. The unit of width and binning is AA-1.
1import matplotlib.pyplot as plt
2from DMCpy import DataSet,DataFile,_tools
3import numpy as np
4
5# Give file number and folder the file is stored in.
6scanNumbers = '12153-12154'
7folder = 'data/SC'
8year = 2022
9
10filePath = _tools.fileListGenerator(scanNumbers,folder,year=year)
11
12unitCell = np.array([ 7.218, 7.218, 18.183, 90.0, 90.0, 120.0])
13
14# # # load dataFiles
15dataFiles = [DataFile.loadDataFile(dFP,unitCell = unitCell) for dFP in filePath]
16
17# load data files and make data set
18ds = DataSet.DataSet(dataFiles)
19
20# Define Q coordinates and HKL for the coordinates.
21q1 = [-0.447,-0.914,-0.003]
22q2 = [-1.02,-0.067,-0.02]
23HKL1 = [1,0,0]
24HKL2 = [0,1,0]
25
26# this function uses two coordinates in Q space and align them to corrdinates in HKL space
27ds.alignToRefs(q1=q1,q2=q2,HKL1=HKL1,HKL2=HKL2)
28
29# define 2D cut width orthogonal to cut plane
30width = 0.5
31
32# these points define the plane that will be cut
33points = np.array([[0.0,0.0,0.0],
34 [1.0,0.0,0.0],
35 [0.0,1.0,0.0]])
36
37kwargs = {
38 'dQx' : 0.01,
39 'dQy' : 0.01,
40 'steps' : 151,
41 'rlu' : True,
42 'rmcFile' : True,
43 'colorbar' : True,
44 }
45
46ax,returndata,bins = ds.plotQPlane(points=points,width=width,**kwargs)
47
48ax.set_clim(0,0.0001)
49
50planeFigName = 'docs/Tutorials/2Dcut_hk0'
51plt.savefig('figure0.png',format='png')
52
53# save csv and txt file with data
54if False:
55 kwargs = {
56 'rmcFileName' : planeFigName+'.txt'
57 }
58
59 ax.to_csv(planeFigName+'.csv',**kwargs)
60
61
62# cut 2D plane orhtogonal to scattering plane
63width = 0.5
64
65points = np.array([[0.0,0.0,0.0],
66 [-1.0,-2.0,0.0],
67 [0.0,0.0,1.0]])
68
69kwargs = {
70 'dQx' : 0.01,
71 'dQy' : 0.01,
72 'steps' : 151,
73 'rlu' : True,
74 'rmcFile' : True,
75 'colorbar' : True,
76 }
77
78ax,returndata,bins = ds.plotQPlane(points=points,width=width,**kwargs)
79
80ax.set_clim(0,0.0001)
81ax.set_xticks_number(7)
82ax.set_yticks_number(3)
83
84ax.colorbar.set_label('')
85ax.colorbar.remove()
86plt.gcf().colorbar(ax.colorbar.mappable,ax=ax,orientation='horizontal', location='top')
87
88planeFigName = 'docs/Tutorials/2Dcut_hk0_side'
89plt.savefig('figure1.png',format='png')
90
91if False:
92 kwargs = {
93 'rmcFileName' : planeFigName+'.txt'
94 }
95
96 ax.to_csv(planeFigName+'.csv',**kwargs)
The above code takes the data from the A3 scan files dmc2022n012153-dmc2022n012154, align and plot the scattering plane.
Figure of the 2D plane in RLU.
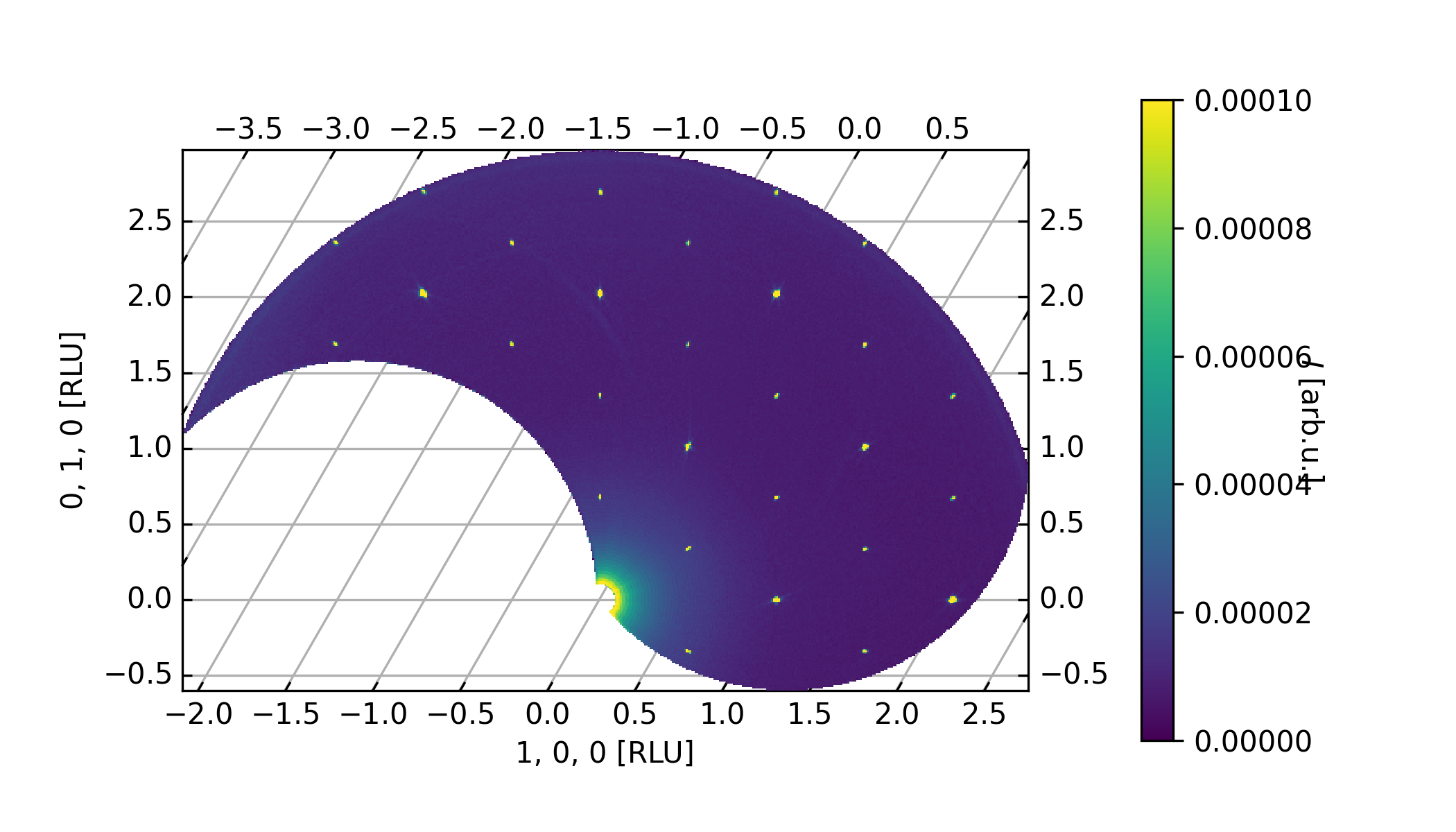
Figure of the 2D plane in RLU.
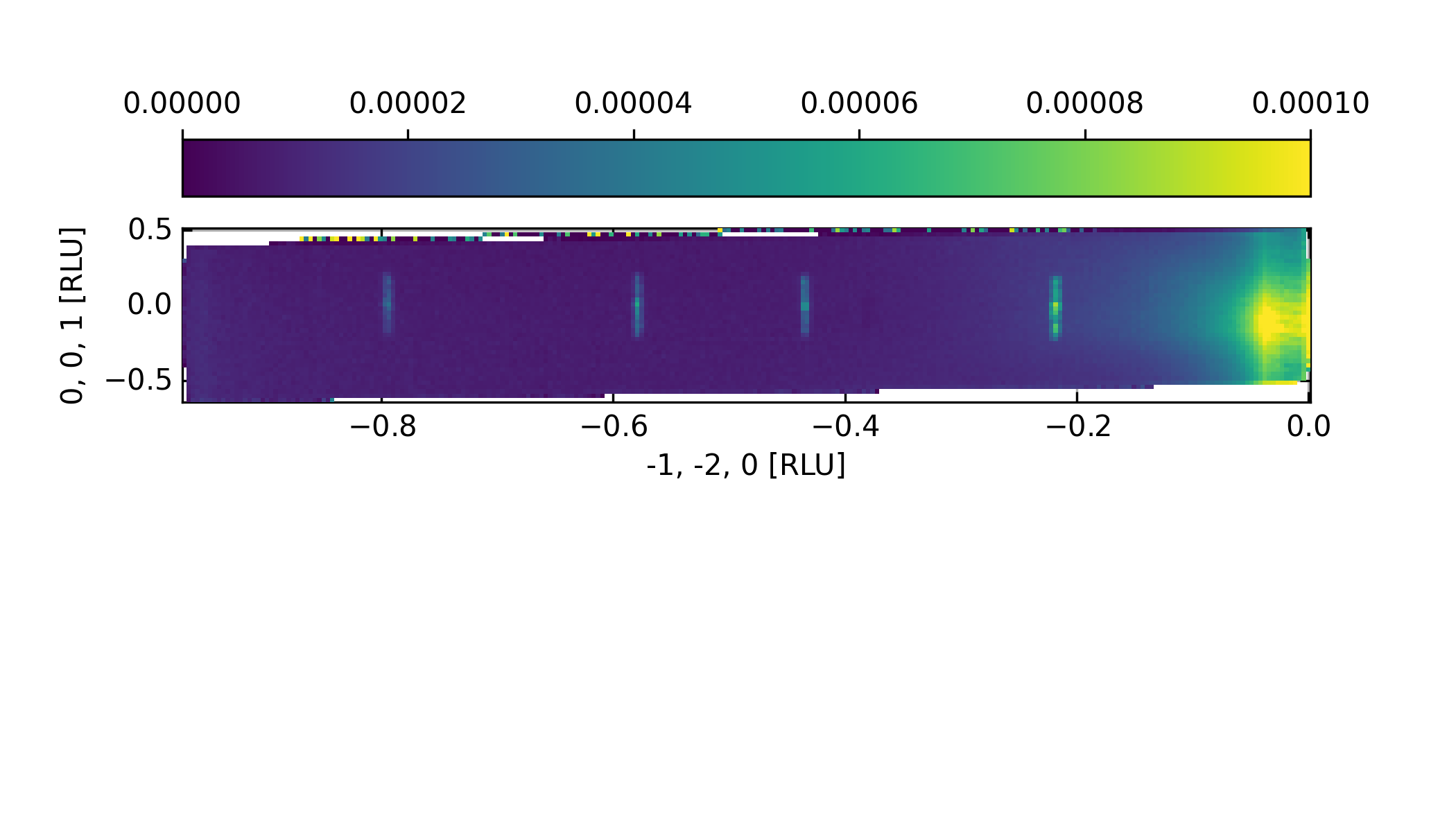